-
Notifications
You must be signed in to change notification settings - Fork 22
getting start
Getting POCO class Model with control of code generation from OData feeds has never been easier than using OData2Poco Commandline tool (a.k.a o2pgen.exe).
OData2Poco CLI allows you to create POCO classes in just a few seconds.
The instructions contained here will help you to use OData2Poco commandline (o2pgen.exe) in short time and with little effort.
OData2Poco.CommandLine (aka o2pgen.exe) is a console tool. This package has no dependencies on other dll and There are no framework assets in this package. It can be referenced in FullDotNet Frameworks net45..net48 , netstandard2.x and NetCore: netcoreapp3.1,net5,net6 and net7.
It can be installed and executed in different ways.
- Download the latest nupkg from OData2Poco.CommandLine on NuGet.org
- Click
Download package
link. - Extract tools/o2pgen.exe to a local folder and run it.
You can use the next powershell code to automate downloading and extract o2pgen.exe
param ($version)
if ($version -eq $null){
$url="https://www.nuget.org/api/v2/package/OData2Poco.CommandLine"
}
else {
$url="https://www.nuget.org/api/v2/package/OData2Poco.CommandLine/$version"
}
$tempFilePath = Join-Path $env:temp 'OData2Poco.CommandLine.nupkg'
#Invoke-RestMethod -Uri $url -OutFile $tempFilePath
curl.exe -s -L -o $tempFilePath -L $url
7z e -y $tempFilePath '-i!tools\o2pgen.exe'
& ./o2pgen.exe --version
NOTE: Also you can download o2pgen.exe from github Release at: https://github.com/moh-hassan/odata2poco/releases
nuget.exe install OData2Poco.CommandLine -ExcludeVersion
Run OData2Poco.CommandLine/tools/o2pgen.exe You can add its path to PATH.
-
Instal the package into the project. The package has on dependency on other dll files. It's considered as a development tool.
-
In the Package Console Manager,run the command:
Install-Package OData2Poco.CommandLine
--or--
dotnet add package OData2Poco.CommandLine
Nuget will install the recent package and Visual studio will add the next xml code in the <project>.csproj
:
<PackageReference Include="OData2Poco.CommandLine" Version="5.1.0">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
</PackageReference>
Note: You can add this xml code directly in your project.
The package contain Cli console tool named o2pgen.exe and it's installed in the package cache folder.
Within the project, you can Execute o2pgen.exe in two different ways:
A) Executing o2pgen.exe from a target in the project:
O2pgen.exe can be executed as MsBuild Target. Add the next xml code to the project .csproj:
<Target Name="Odata2PocoRun" BeforeTargets="Build" >
<PropertyGroup>
<EnableCodeGeneration>true</EnableCodeGeneration>
<o2pgen>$(PkgOData2Poco_CommandLine)\tools\o2pgen.exe</o2pgen>
<options>-r http://services.odata.org/V4/Northwind/Northwind.svc/ -f Model\north.cs</options>
</PropertyGroup>
<Message Text="Executing o2pgen.exe" Importance="High" />
<Exec Condition="$(EnableCodeGeneration)
Command="$(o2pgen) $(options)" />
</Target>
The attribute EnableCodeGeneration
is true, so target is executed before build.
To stop the excution of the tool set EnableCodeGeneration
to false
.
The attribute Options
is the commandLine arguments. Modify the commandline options as you want.
You can review the output in the output
tab in visual studio, or in terminal for VS code IDE.
When Visual studio install the package, it add the path of o2pgen to the path of PMC and you can call the tool as:
PM> o2pgen <options>
Check the installation by running the command:
PM> o2pgen --version
output:
O2Pgen (net472) 5.0.1+10ddf8bc1
From terminal you can run o2pgen as described in Visual Studio.
Generating the model with minimal (no configuration):
To generate POCO classes for the service "http://services.odata.org/V4/Northwind/Northwind.svc", type the following command:
o2pgen -r http://services.odata.org/V4/Northwind/Northwind.svc/
Now, you find the following text in the console:
Start processing url: http://services.odata.org/V4/Northwind/Northwind.svc/
Saving generated code to file : poco.cs
Total processing time: 1.261 sec (time may be different)
The command generate a complete CSharp model for the OData service and save the code in a file named poco.cs (default file name, but you can control name and location )
You can review the generated code Default model
You can include the model in your project and build your API-client application using the POCO typed classes.
The generated model haven't navigation properties of the class. For example: Category class have no property for the Product class
Mono should be installed. For mono installation read.
Run the command:
mono O2Pgen.exe --lang CS --url http://services.odata.org/V4/OData/OData.svc
Generating POCO model including navigation properties
Type the following command by adding the option -n
o2pgen -r http://services.odata.org/V4/Northwind/Northwind.svc/ -n
or you can merge the options as below:
o2pgen -nr http://services.odata.org/V4/Northwind/Northwind.svc/
It's simple. Is it?
The next sections describe how to control code generation based on your needs and model usage.
- To filter the model and narrow the generated classes, see --include
- All commandline Options
dotnet Global tool (OData2Poco.dotnet.o2pgen) is available. Requirments: net6 runtime. It can run in Windows /Linux and MacOs
You can install by runing the command:
dotnet tool install --global OData2Poco.dotnet.o2pgen
To use, you can run :
dotnet o2pgen -r http://services.odata.org/V4/Northwind/Northwind.svc -v
You have the same options of the console tool: o2pgen.exe
You can auto run dotnet o2pgen
from within MsBuild Task and save code in the project folder.
Add the next Msbuild target to your project and modify command parameters as needed.
When the property EnableCodeGeneration
is set to false
, no code is generated.
The generated code is saved to file northwind.cs
in the folder Model in the root of the project.
<Target Name="GenerateCode" BeforeTargets="Build">
<PropertyGroup>
<EnableCodeGeneration>true</EnableCodeGeneration>
</PropertyGroup>
<Exec Condition="$(EnableCodeGeneration)"
Command="dotnet o2pgen -r http://services.odata.org/V4/Northwind/Northwind.svc/ -f $(MSBuildProjectDirectory)\Model\northwind.cs -B">
</Exec>
</Target>
** 3) Install o2pgen from Chocolatey Gallery** O2pgen can be used as a separate tool without project. To install, run the following command from cmd window shell:
choco install odata2poco-commandline
choco add o2pgen.exe to the path of the machine, and you can run it as:
o2pgen <options>
Show screen capture:
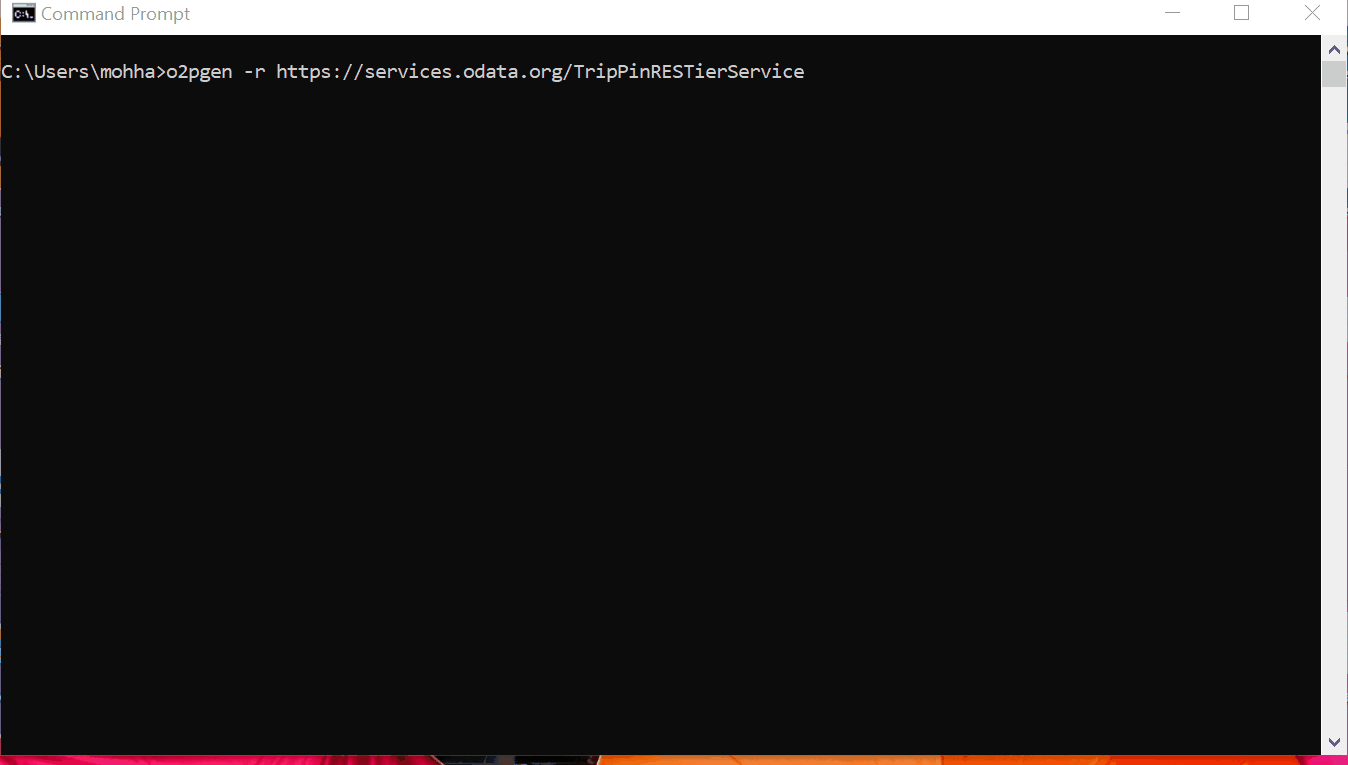
- home
- Announcing V6.0.0
- Features
- Getting started with c# generation
- Http Connection
- Using Parameter file
- User Defined Attributes
- Controlling c# code generation
- Model Filter
- Enable Nullable Reference type of c# 8
- Class with Init-Only Properties (c# 9)
- Generating Constructor
- Record-Type (c# 9)
- Name Map
- Securing Password
- Using Proxy Server
- Using Plugin Attributes
- Developing with OData2Poco
- Examples in dotnetfiddle.net
- CommandLine-Reference
- AttributeExamples
- typescript generation
- Help Screen
- How to
- New Feature 4.2.1
Samples of generated code: