-
-
Notifications
You must be signed in to change notification settings - Fork 233
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Use pty with no command? #153
Comments
Hi! I was tackling a similar problem and creack's pty might be a bit too abstracted for what you want. I just used the golang standard xterm terminal emulator https://pkg.go.dev/golang.org/x/term and a pipe. Here's a partial snippet as an example of what I mean. Your stdin stream is fed into the
|
Oh wow, thank you so much for this info @lgmugnier ! Somehow never came across the golang xterm emulator, this seems very likely to be exactly what I need... If you copy/paste the above to my according stack overflow question here, it might reach more folks and I'd be happy to accept it as the answer for some internet points for you |
It took me a while to find it as well
already done : ) |
Thanks @creack for the great library and community support!
I have a question that I hope makes sense.
I am using a websocket api that sends stdin messages and receives stdout and stderr output from the command as well as exit codes.
For example, this API is used in a web UI, with the following string of messages sent and received
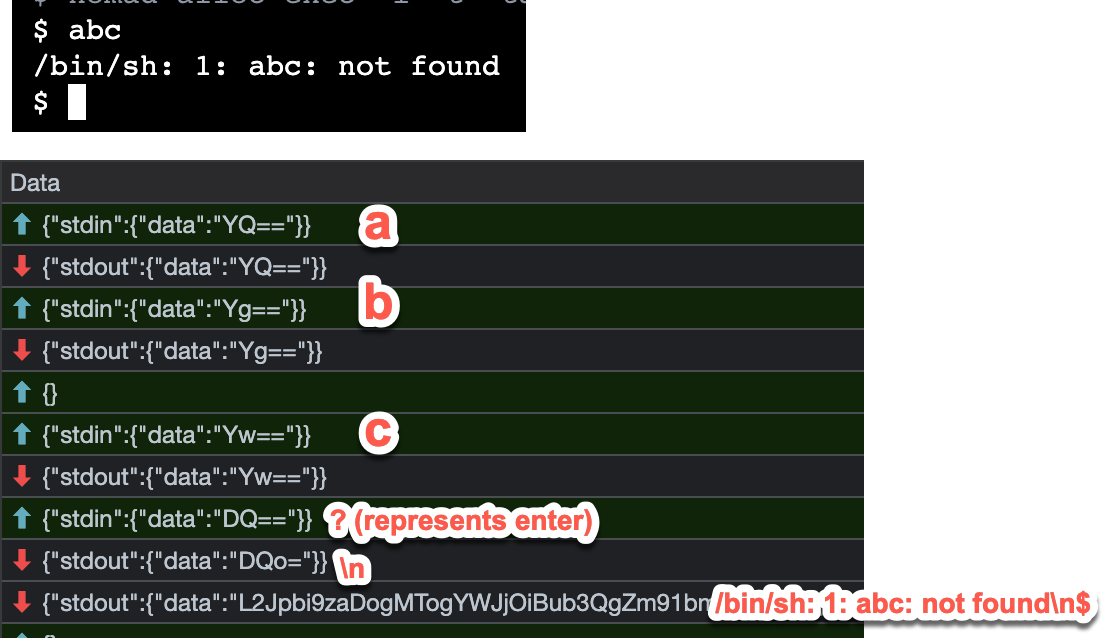
This web UI uses xterm.js to provide a terminal-like input ui and to interpret the responses, including ansi escape sequences, into terminal output.
I am building a terminal application that would like to leverage this same API, so a "terminal-in-a-terminal" like thing, where stdin is sent to the API and responses received are rendered in my application.
I would like to use creack/pty as the response interpreter, handling ansi escape sequences and the like, and holding a view of the terminal session that I can read into a string and render to the screen of my application.
So the flow is roughly like:
If I use creack/pty this way, I don't actually have a command to start - it's just a nice box that interprets ansi escape sequences for me and allows me to retrieve the current "string view" of the terminal.
Here is my attempt to get a command-less pty, write to it, and read from it:
I get the following error
Is what I'm trying to do sane at all? Is there a better way to achieve my goal here?
Thank you!
Leo
The text was updated successfully, but these errors were encountered: